There are two main modules in Python that deal with path manipulation. One is the os.path
module and the other is the pathlib
module.
os.path VS pathlib
The `pathlib` module was added in Python 3.4, offering an object-oriented way to handle file system paths.
Linux and Windows Paths
On Windows, paths are written using backslashes (\
) as the separator between folder names. On Unix based operating system such as macOS, Linux, and BSDs, the forward slash (/
) is used as the path separator. Joining paths can be a headache if your code needs to work on different platforms.
Fortunately, Python provides easy ways to handle this. We will showcase how to deal with both, os.path.join
and pathlib.Path.joinpath
Using os.path.join
on Windows:
>>> import os
>>> os.path.join('usr', 'bin', 'spam')
# 'usr\\bin\\spam'
And using pathlib
on *nix:
>>> from pathlib import Path
>>> print(Path('usr').joinpath('bin').joinpath('spam'))
# usr/bin/spam
pathlib
also provides a shortcut to joinpath using the /
operator:
>>> from pathlib import Path
>>> print(Path('usr') / 'bin' / 'spam')
# usr/bin/spam
Notice the path separator is different between Windows and Unix based operating system, that’s why you want to use one of the above methods instead of adding strings together to join paths together.
Joining paths is helpful if you need to create different file paths under the same directory.
Using os.path.join
on Windows:
>>> my_files = ['accounts.txt', 'details.csv', 'invite.docx']
>>> for filename in my_files:
... print(os.path.join('C:\\Users\\asweigart', filename))
...
# C:\Users\asweigart\accounts.txt
# C:\Users\asweigart\details.csv
# C:\Users\asweigart\invite.docx
Using pathlib
on *nix:
>>> my_files = ['accounts.txt', 'details.csv', 'invite.docx']
>>> home = Path.home()
>>> for filename in my_files:
... print(home / filename)
...
# /home/asweigart/accounts.txt
# /home/asweigart/details.csv
# /home/asweigart/invite.docx
The current working directory
Using os
on Windows:
>>> import os
>>> os.getcwd()
# 'C:\\Python34'
>>> os.chdir('C:\\Windows\\System32')
>>> os.getcwd()
# 'C:\\Windows\\System32'
Using pathlib
on *nix:
>>> from pathlib import Path
>>> from os import chdir
>>> print(Path.cwd())
# /home/asweigart
>>> chdir('/usr/lib/python3.6')
>>> print(Path.cwd())
# /usr/lib/python3.6
Creating new folders
Using os
on Windows:
>>> import os
>>> os.makedirs('C:\\delicious\\walnut\\waffles')
Using pathlib
on *nix:
>>> from pathlib import Path
>>> cwd = Path.cwd()
>>> (cwd / 'delicious' / 'walnut' / 'waffles').mkdir()
# Traceback (most recent call last):
# File "<stdin>", line 1, in <module>
# File "/usr/lib/python3.6/pathlib.py", line 1226, in mkdir
# self._accessor.mkdir(self, mode)
# File "/usr/lib/python3.6/pathlib.py", line 387, in wrapped
# return strfunc(str(pathobj), *args)
# FileNotFoundError: [Errno 2] No such file or directory: '/home/asweigart/delicious/walnut/waffles'
Oh no, we got a nasty error! The reason is that the ‘delicious’ directory does not exist, so we cannot make the ‘walnut’ and the ‘waffles’ directories under it. To fix this, do:
>>> from pathlib import Path
>>> cwd = Path.cwd()
>>> (cwd / 'delicious' / 'walnut' / 'waffles').mkdir(parents=True)
And all is good
Absolute vs. Relative paths
There are two ways to specify a file path.
- An absolute path, which always begins with the root folder
- A relative path, which is relative to the program’s current working directory
There are also the dot (.
) and dot-dot (..
) folders. These are not real folders, but special names that can be used in a path. A single period (“dot”) for a folder name is shorthand for “this directory.” Two periods (“dot-dot”) means “the parent folder.”
Handling Absolute paths
To see if a path is an absolute path:
Using os.path
on *nix:
>>> import os
>>> os.path.isabs('/')
# True
>>> os.path.isabs('..')
# False
Using pathlib
on *nix:
>>> from pathlib import Path
>>> Path('/').is_absolute()
# True
>>> Path('..').is_absolute()
# False
You can extract an absolute path with both os.path
and pathlib
Using os.path
on *nix:
>>> import os
>>> os.getcwd()
'/home/asweigart'
>>> os.path.abspath('..')
'/home'
Using pathlib
on *nix:
from pathlib import Path
print(Path.cwd())
# /home/asweigart
print(Path('..').resolve())
# /home
Handling Relative paths
You can get a relative path from a starting path to another path.
Using os.path
on *nix:
>>> import os
>>> os.path.relpath('/etc/passwd', '/')
# 'etc/passwd'
Using pathlib
on *nix:
>>> from pathlib import Path
>>> print(Path('/etc/passwd').relative_to('/'))
# etc/passwd
Path and File validity
Checking if a file/directory exists
Using os.path
on *nix:
>>> import os
>>> os.path.exists('.')
# True
>>> os.path.exists('setup.py')
# True
>>> os.path.exists('/etc')
# True
>>> os.path.exists('nonexistentfile')
# False
Using pathlib
on *nix:
from pathlib import Path
>>> Path('.').exists()
# True
>>> Path('setup.py').exists()
# True
>>> Path('/etc').exists()
# True
>>> Path('nonexistentfile').exists()
# False
Checking if a path is a file
Using os.path
on *nix:
>>> import os
>>> os.path.isfile('setup.py')
# True
>>> os.path.isfile('/home')
# False
>>> os.path.isfile('nonexistentfile')
# False
Using pathlib
on *nix:
>>> from pathlib import Path
>>> Path('setup.py').is_file()
# True
>>> Path('/home').is_file()
# False
>>> Path('nonexistentfile').is_file()
# False
Checking if a path is a directory
Using os.path
on *nix:
>>> import os
>>> os.path.isdir('/')
# True
>>> os.path.isdir('setup.py')
# False
>>> os.path.isdir('/spam')
# False
Using pathlib
on *nix:
>>> from pathlib import Path
>>> Path('/').is_dir()
# True
>>> Path('setup.py').is_dir()
# False
>>> Path('/spam').is_dir()
# False
Getting a file’s size in bytes
Using os.path
on Windows:
>>> import os
>>> os.path.getsize('C:\\Windows\\System32\\calc.exe')
# 776192
Using pathlib
on *nix:
>>> from pathlib import Path
>>> stat = Path('/bin/python3.6').stat()
>>> print(stat) # stat contains some other information about the file as well
# os.stat_result(st_mode=33261, st_ino=141087, st_dev=2051, st_nlink=2, st_uid=0,
# --snip--
# st_gid=0, st_size=10024, st_atime=1517725562, st_mtime=1515119809, st_ctime=1517261276)
>>> print(stat.st_size) # size in bytes
# 10024
Listing directories
Listing directory contents using os.listdir
on Windows:
>>> import os
>>> os.listdir('C:\\Windows\\System32')
# ['0409', '12520437.cpx', '12520850.cpx', '5U877.ax', 'aaclient.dll',
# --snip--
# 'xwtpdui.dll', 'xwtpw32.dll', 'zh-CN', 'zh-HK', 'zh-TW', 'zipfldr.dll']
Listing directory contents using pathlib
on *nix:
>>> from pathlib import Path
>>> for f in Path('/usr/bin').iterdir():
... print(f)
...
# ...
# /usr/bin/tiff2rgba
# /usr/bin/iconv
# /usr/bin/ldd
# /usr/bin/cache_restore
# /usr/bin/udiskie
# /usr/bin/unix2dos
# /usr/bin/t1reencode
# /usr/bin/epstopdf
# /usr/bin/idle3
# ...
Directory file sizes
WARNING
Directories themselves also have a size! So, you might want to check for whether a path is a file or directory using the methods in the methods discussed in the above section.
Using os.path.getsize()
and os.listdir()
together on Windows:
>>> import os
>>> total_size = 0
>>> for filename in os.listdir('C:\\Windows\\System32'):
... total_size = total_size + os.path.getsize(os.path.join('C:\\Windows\\System32', filename))
...
>>> print(total_size)
# 1117846456
Using pathlib
on *nix:
>>> from pathlib import Path
>>> total_size = 0
>>> for sub_path in Path('/usr/bin').iterdir():
... total_size += sub_path.stat().st_size
...
>>> print(total_size)
# 1903178911
Copying files and folders
The shutil
module provides functions for copying files, as well as entire folders.
>>> import shutil, os
>>> os.chdir('C:\\')
>>> shutil.copy('C:\\spam.txt', 'C:\\delicious')
# C:\\delicious\\spam.txt'
>>> shutil.copy('eggs.txt', 'C:\\delicious\\eggs2.txt')
# 'C:\\delicious\\eggs2.txt'
While shutil.copy()
will copy a single file, shutil.copytree()
will copy an entire folder and every folder and file contained in it:
>>> import shutil, os
>>> os.chdir('C:\\')
>>> shutil.copytree('C:\\bacon', 'C:\\bacon_backup')
# 'C:\\bacon_backup'
Moving and Renaming
>>> import shutil
>>> shutil.move('C:\\bacon.txt', 'C:\\eggs')
# 'C:\\eggs\\bacon.txt'
The destination path can also specify a filename. In the following example, the source file is moved and renamed:
>>> shutil.move('C:\\bacon.txt', 'C:\\eggs\\new_bacon.txt')
# 'C:\\eggs\\new_bacon.txt'
If there is no eggs folder, then move()
will rename bacon.txt to a file named eggs:
>>> shutil.move('C:\\bacon.txt', 'C:\\eggs')
# 'C:\\eggs'
Deleting files and folders
-
Calling
os.unlink(path)
orPath.unlink()
will delete the file at path. -
Calling
os.rmdir(path)
orPath.rmdir()
will delete the folder at path. This folder must be empty of any files or folders. -
Calling
shutil.rmtree(path)
will remove the folder at path, and all files and folders it contains will also be deleted.
Walking a Directory Tree
>>> import os
>>>
>>> for folder_name, subfolders, filenames in os.walk('C:\\delicious'):
... print(f'The current folder is {folder_name}')
... for subfolder in subfolders:
... print(f'SUBFOLDER OF {folder_name}: {subfolder}')
... for filename in filenames:
... print(f'FILE INSIDE {folder_name}: {filename}')
... print('')
...
# The current folder is C:\delicious
# SUBFOLDER OF C:\delicious: cats
# SUBFOLDER OF C:\delicious: walnut
# FILE INSIDE C:\delicious: spam.txt
# The current folder is C:\delicious\cats
# FILE INSIDE C:\delicious\cats: catnames.txt
# FILE INSIDE C:\delicious\cats: zophie.jpg
# The current folder is C:\delicious\walnut
# SUBFOLDER OF C:\delicious\walnut: waffles
# The current folder is C:\delicious\walnut\waffles
# FILE INSIDE C:\delicious\walnut\waffles: butter.txt
Pathlib vs Os Module
`pathlib` provides a lot more functionality than the ones listed above, like getting file name, getting file extension, reading/writing a file without manually opening it, etc. See the official documentation if you intend to know more.
Subscribe to pythoncheatsheet.org
Join 14,100+ Python developers in a two times a month and bullshit free publication , full of interesting, relevant links.
Пройдите тест, узнайте какой профессии подходите
Работать самостоятельно и не зависеть от других
Работать в команде и рассчитывать на помощь коллег
Организовывать и контролировать процесс работы
Введение в работу с путями файлов в Python
Работа с путями файлов является важным аспектом программирования на Python, особенно когда речь идет о манипуляции файлами и директориями. В Python существует несколько модулей, которые помогают упростить эту задачу, включая os
и pathlib
. В этой статье мы рассмотрим основные методы и функции, которые помогут вам эффективно работать с путями файлов в Python. Понимание того, как правильно работать с путями файлов, может значительно упростить вашу работу и сделать ваш код более надежным и переносимым.
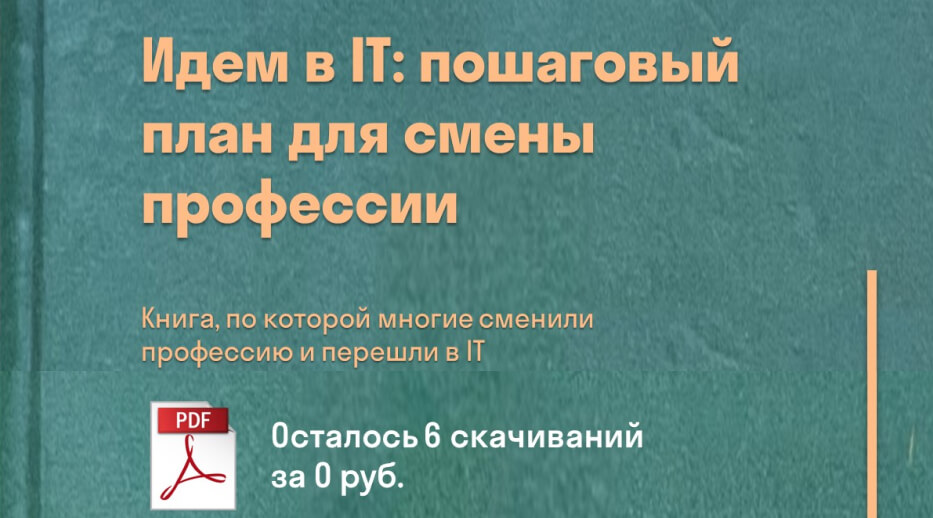
Использование модуля os для работы с путями
Модуль os
предоставляет множество функций для работы с операционной системой, включая функции для работы с путями файлов. Этот модуль является частью стандартной библиотеки Python и широко используется для выполнения различных задач, связанных с операционной системой. Вот некоторые из наиболее часто используемых функций:
os.path.join
Функция os.path.join
используется для объединения нескольких компонентов пути в один путь. Это особенно полезно для создания путей, которые будут работать на разных операционных системах. Например, на Unix-системах разделителем путей является /
, а на Windows — \
. Использование os.path.join
позволяет избежать проблем с несовместимостью путей.
os.path.exists
Функция os.path.exists
проверяет, существует ли указанный путь. Это полезно для проверки наличия файла или директории перед выполнением операций, которые могут вызвать ошибки, если путь не существует.
os.path.abspath
Функция os.path.abspath
возвращает абсолютный путь для указанного относительного пути. Это полезно для получения полного пути к файлу или директории, что может быть необходимо для выполнения определенных операций.
Преимущества и использование модуля pathlib
Модуль pathlib
был введен в Python 3.4 и предоставляет объектно-ориентированный подход к работе с путями файлов. Он обладает рядом преимуществ по сравнению с модулем os
, таких как более интуитивный и читаемый синтаксис, а также поддержка всех основных операций с путями файлов.
Создание пути
Создание пути с использованием pathlib
выглядит более интуитивно и читаемо. Вместо использования строк и функций, вы работаете с объектами, что делает код более понятным и легким для чтения.
Проверка существования пути
Проверка существования пути с использованием pathlib
также проста и интуитивна. Вместо вызова функции, вы просто вызываете метод объекта.
Получение абсолютного пути
Получение абсолютного пути с использованием pathlib
выполняется с помощью метода resolve
. Этот метод возвращает полный путь к файлу или директории.
Получение текущего пути и навигация по файловой системе
Работа с текущим путем и навигация по файловой системе является важной частью работы с путями файлов. Рассмотрим, как это можно сделать с использованием модулей os
и pathlib
.
Получение текущего пути
С помощью модуля os
можно получить текущий рабочий каталог с помощью функции os.getcwd
. Это полезно для определения текущего местоположения в файловой системе.
С помощью модуля pathlib
можно получить текущий рабочий каталог с помощью метода Path.cwd
. Этот метод возвращает объект Path, представляющий текущий рабочий каталог.
Навигация по файловой системе
Навигация по файловой системе может включать переход в другой каталог, создание новых директорий и удаление существующих. Эти операции могут быть выполнены с использованием как модуля os
, так и модуля pathlib
.
Переход в другой каталог
С помощью модуля os
можно изменить текущий рабочий каталог с помощью функции os.chdir
. Это полезно для выполнения операций в другом каталоге.
С помощью модуля pathlib
можно изменить текущий рабочий каталог с помощью метода chdir
. Этот метод изменяет текущий рабочий каталог на указанный путь.
Создание новой директории
С помощью модуля os
можно создать новую директорию с помощью функции os.makedirs
. Эта функция создает все промежуточные директории, если они не существуют.
С помощью модуля pathlib
можно создать новую директорию с помощью метода mkdir
. Этот метод также может создавать все промежуточные директории, если указать параметр parents=True
.
Удаление директории
С помощью модуля os
можно удалить директорию с помощью функции os.rmdir
. Эта функция удаляет только пустые директории.
С помощью модуля pathlib
можно удалить директорию с помощью метода rmdir
. Этот метод также удаляет только пустые директории.
Практические примеры и советы
Пример 1: Создание и удаление файла
Создание и удаление файла является одной из основных операций при работе с путями файлов. Рассмотрим, как это можно сделать с использованием pathlib
.
Пример 2: Чтение и запись в файл
Чтение и запись в файл также являются важными операциями. Рассмотрим, как это можно сделать с использованием pathlib
.
Совет: Используйте pathlib для новых проектов
Если вы начинаете новый проект или обновляете существующий, рекомендуется использовать модуль pathlib
вместо os
для работы с путями файлов. pathlib
предоставляет более удобный и интуитивно понятный интерфейс, а также поддерживает все основные операции, необходимые для работы с путями файлов. Это делает код более читаемым и легким для сопровождения.
Работа с путями файлов в Python может быть простой и эффективной, если использовать правильные инструменты. Модули os
и pathlib
предоставляют все необходимые функции для выполнения различных операций с путями файлов, и выбор между ними зависит от ваших предпочтений и требований проекта. Независимо от того, какой модуль вы выберете, важно понимать основные концепции и методы работы с путями файлов, чтобы ваш код был надежным и переносимым.
Читайте также
On this page: open(), file path, CWD (‘current working directory’), r ‘raw string’ prefix, os.getcwd(), os.chdir().
Referencing a File with a Full Path and Name
As seen in Tutorials #12 and #13, you can refer to a local file in Python using the file’s full path and file name. Below, you are opening up a file for reading:
>>> myfile = open('C:/Users/yourname/Desktop/alice.txt') >>> mytxt = myfile.read() >>> myfile.close() |
>>> myfile = open('/Users/yourname/Desktop/alice.txt') >>> mytxt = myfile.read() >>> myfile.close() |
In Windows, a full file directory path starts with a drive letter (C:, D:. etc.). In Linux and OS-X, it starts with «/», which is called root. Directories are separated by a slash «/». You can look up a file’s full directory path and file name through its «Properties». See how it is done in this FAQ.
Referencing a File in Windows
In Windows, there are a couple additional ways of referencing a file. That is because natively, Windows file path employs the backslash «\» instead of the slash. Python allows using both in a Windows system, but there are a couple of pitfalls to watch out for. To sum them up:
- Python lets you use OS-X/Linux style slashes «/» even in Windows. Therefore, you can refer to the file as ‘C:/Users/yourname/Desktop/alice.txt’. RECOMMENDED.
- If using backslash, because it is a special character in Python, you must remember to escape every instance: ‘C:\\Users\\yourname\\Desktop\\alice.txt’
- Alternatively, you can prefix the entire file name string with the rawstring marker «r»: r’C:\Users\yourname\Desktop\alice.txt’. That way, everything in the string is interpreted as a literal character, and you don’t have to escape every backslash.
File Name Shortcuts and CWD (Current Working Directory)
So, using the full directory path and file name always works; you should be using this method. However, you might have seen files called by their name only, e.g., ‘alice.txt’ in Python. How is it done?
The concept of Current Working Directory (CWD) is crucial here. You can think of it as the folder your Python is operating inside at the moment. So far we have been using the absolute path, which begins from the topmost directory. But if your file reference does not start from the top (e.g.,
‘alice.txt’
,
‘ling1330/alice.txt’
), Python assumes that it starts in the CWD (a «relative path«).
This means that a name-only reference will be successful only when the file is in your Python’s CWD. But bear in mind that your CWD may change. Also, your Python has different initial CWD settings depending on whether you are working with a Python script or in a shell environment.
- In a Python script:
When you execute your script, your CWD is set to the directory where your script is. Therefore, you can refer to a file in a script by its name only provided that the file and the script are in the same directory. An example:myfile = open('alice.txt') mytxt = myfile.read() myfile.close() foo.py
- In Python shell:
In your shell, the initial CWD setting varies by system. In Windows, the default location is often ‘C:/program Files (x86)/Python35-32’ (which is inconvenient — see this «Basic Configurations» page or this FAQ for how to change it). In OS-X, it is usually ‘/Users/username/Documents’ where username is your user ID. (Mac users should see this FAQ for how to change your setting.)Unless your file happens to be in your CWD, you have two options:
- Change your CWD to the file’s directory, or
- Copy or move your file to your CWD. (Not recommended, since your shell’s CWD may change.)
See this screen shot and and the next section for how to work with your CWD setting in Python shell.
Finding and Changing CWD
Python module os provides utilities for displaying and modifying your current working directory. Below illustrates how to find your CWD (.getcwd()) and change it into a different directory (.chdir()). Below is an example for the windows OS:
>>> import os >>> os.getcwd() 'D:\\Lab' >>> os.chdir('scripts/gutenberg') >>> os.getcwd() 'D:\\Lab\\scripts\\gutenberg' >>> os.chdir(r'D:\Corpora\corpus_samples') >>> os.getcwd() 'D:\\Corpora\\corpus_samples' |
Note that the CWD returned by Python interpreter is in the Windows file path format: it uses the backslash «\» for directory separator, and every instance is escaped. While Python lets Windows users use Linux/OS-X style «/» in file paths, internally it uses the OS-native file path format.
Взаимодействие с файловой системой#
Нередко требуется программными средствами взаимодействовать с файловой системой и в стандартной библиотеке python
реализовано много инструментов, значительно упрощающих этот процесс.
Путь к файлу/директории#
Путь (англ. path) — набор символов, показывающий расположение файла или каталога в файловой системе (источник — wikipedia). В программных средах путь необходим, например, для того, чтобы открывать и сохранять файлы. В большинстве случаев в python
путь представляется в виде обычного строкового объекта.
Обычно путь представляет собой последовательность вложенных каталогов, разделенных специальным символом, при этом разделитель каталогов может меняться в зависимости от операционной системы: в OS Windows
используется “\
”, в unix-like
системах — “/
”. Кроме того, важно знать, что пути бывают абсолютными и относительными. Абсолютный путь всегда начинается с корневого каталога файловой системы (в OS Windows
— это логический раздел (например, “C:”), в UNIX-like
системах — “/”) и всегда указывает на один и тот же файл (или директорию). Относительный путь, наоборот, не начинается с корневого каталога и указывает расположение относительно текущего рабочего каталога, а значит будет указывать на совершено другой файл, если поменять рабочий каталог.
Итого, например, путь к файлу “hello.py” в домашней директории пользователя “ivan” в зависимости от операционной системы будет выглядеть приблизительно следующим образом:
|
|
|
---|---|---|
Глобальный |
C:\Users\ivan\hello.py |
/home/users/ivan/hello.py |
Относительный |
.\hello.py |
./hello.py |
В связи с этим требуется прикладывать дополнительные усилия, чтобы заставить работать один и тот же код на машинах с разными операционными системами. Чтобы все же абстрагироваться от того, как конкретно устроена файловая система на каждой конкретной машине, в python
предусмотренны модули стандартной библиотеки os.path и pathlib.
Проблема с путями в стиле Windows
#
Как было отмечено выше, в Windows
в качестве разделителя используется символ обратного слеша (backslash) “\
”. Это может привести к небольшой путанице у неопытных программистов. Дело в том, что во многих языка программирования (и в python
, в том числе) символ “\
” внутри строк зарезервирован для экранирования, т.е. если внутри строки встречается “
“, то он интерпретируется не буквально как символ обратного слеша, а изменяет смысл следующего за ним символом. Так, например, последовательность "\n"
представляет собой один управляющий символ перевода строки.
new_line = "\n" print(len(new_line))
Это значит, что если вы попробуете записать Windows
путь не учитывая эту особенность, то высока вероятность получить не тот результат, который вы ожидали. Например, строка "C:\Users"
вообще не корректна с точки зрения синтаксиса python
:
users_folder = "C:\Users"
Input In [10] users_folder = "C:\Users" ^ SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
Это объясняется тем, что последовательность "\U"
используется для экранирования unicode
последовательностей, а набор символов "sers"
не является корректным unicode
кодом. Ниже приводится пример корректного unicode
кода.
snake_emoji = "\U0001F40D" print(snake_emoji)
В python
предусмотренно как минимум два подхода борьбы с этой проблемой.
Первый из них опирается на удвоение количества символов “\
”. Дело в том, что в последовательности символов “\\
” — первый обратный слеш экранирует второй, т.е. итоговый результат эквивалентен одному настоящему символу обратного слеша.
users_folder = "C:\\Users" print(users_folder) new_line = "\\n" print(len(new_line))
Второй способ опирается на использование так называемых сырых (raw) строк: если перед началом литерала строки поставить символ “r
”, то символ обратного слеша теряет свою особую роль внутри неё.
users_folder = r"C:\Users" print(users_folder) new_line = r"\n" print(len(new_line))
Сам факт того, что при ручном прописывании пути в виде строки приходится проявлять дополнительную бдительность намекает на то, что должен быть более осмысленный способ составлении пути.
/
Соединение элементов пути#
Рассмотрим конкретный пример. Пусть у нас имеется строка folder
, представляющая путь к каталогу, и строка filename
, представляющее имя некоего файла внутри этого каталога.
folder = "directory" filename = "file.txt"
Чтобы открыть этот файл, нам потребуется соединить эти две строки, учитывая разделитель каталогов.
Конечно, можно вспомнить, что путь — строка, а значит их можно конкатенировать. Но, что если кто-то захочет запустить ваш код на машине с другой операционной системой? Гораздо целесообразнее воспользоваться для этих целей специальными средствами. Самый надежный способ — метод os.path.join, который на вход принимает произвольное количество имен файлов и соединяет их тем символом, который используется в качестве разделителя на той конкретной машине, на которой скрипт запущен сейчас.
import os path = os.path.join(folder, filename) print(path)
Альтернативой является модуль pathlib, который позволяет обращаться с путями файловой системы в объектно ориентированном стиле, т.е. путь больше не представляется в виде строки, а в виде специального объекта, который в любой момент может быть приведен к строке конструктором строки str.
Для создания такого объекта обычно используют класс Path, при создании экземпляра которого учитывается операционная система, на которой запущен данный скрипт.
from pathlib import Path folder = Path(folder) print(f"{folder=}, {str(folder)=}")
folder=WindowsPath('directory'), str(folder)='directory'
В ячейке выше создается объект типа Path
из строки folder
и вывод сообщает, что создался объект WindowsPath('directory
. Обратите внимание, что автоматически создался путь OS Windows
, т.к. этот скрипт запускался под управлением этой операционной системы.
Чтобы присоединить имя файла к объекту folder
, можно использовать оператор “/
” вне зависимости от операционной системы.
path = folder / filename print(f"{path=}, {str(path)=}")
path=WindowsPath('directory/file.txt'), str(path)='directory\\file.txt'
Обратите внимание на то, что при приведении к строке автоматически получилась строка с разделителем в стиле OS Windows
, т.к. при генерации материалов использовался компьютер под управлением OS Windows
.
Автор курса рекомендует всегда использовать средства модулей os.path или pathlib, даже если вам известно, что ваш скрипт будет запускаться под управлением какой-то конкретной операционной системы, чтобы писать более надежный код и формировать полезные привычки.
Извлечение элементов из пути#
Иногда может стоять обратная задача: дан путь, а из него надо что-то извлечь.
path = r"C:\Users\fadeev\folder\file.txt"
Метод os.path.splitdrive разбивает строку на логический раздел и остальное (актуально в основном на OS Windows
).
print(f"{path=}") drive, tail = os.path.splitdrive(path) print(f"{drive=}, {tail=}")
path='C:\\Users\\fadeev\\folder\\file.txt' drive='C:', tail='\\Users\\fadeev\\folder\\file.txt'
Метод os.path.dirname выделяет из пути родительский каталог.
parent_folder = os.path.dirname(path) print(f"{parent_folder=}")
parent_folder='C:\\Users\\fadeev\\folder'
Метод os.path.basename наоборот извлекает имя файла или папки, на которую данный путь указывает без учета родительского каталога.
filename = os.path.basename(path) print(f"{filename=}")
Метаинформация файла/каталога#
Имея путь, можно запрашивать у операционной системы информацию о том, что находится по этому пути. Важно понимать, что на этом этапе всегда происходит запрос к операционной системе и, если у запустившего программу пользователя не хватает привилегий для выполнения запрошенной операции, то в зависимости от операционной системы вы можете получить разные ответы.
Самый фундаментальный вопрос, который можно задать — существует ли вообще что-нибудь по указанному пути? Метод os.path.exists отвечает как раз на этот вопрос.
print(f"{os.path.exists(path)=}, {os.path.exists('filesystem.ipynb')=}")
os.path.exists(path)=False, os.path.exists('filesystem.ipynb')=True
Методы os.path.isdir и os.path.isfile позволяют определить располагает ли по этому пути каталог или файл соответственно. Оба метода возвращают False
, если по переданному пути ничего не располагается.
print(f"{os.path.isdir(folder)=}, {os.path.isfile('filesystem.ipynb')=}")
os.path.isdir(folder)=True, os.path.isfile('filesystem.ipynb')=True
Также иногда бывает полезно узнать время создания (последнего изменения) или последнего доступа к файлу или каталогу. Для этих целей существуют методы os.path.getatime, os.path.getmtime и os.path.getctime. Размер файла можно узнать методом os.path.getsize.
Содержимое каталога#
В ряде задач может потребоваться узнать содержимое определенного каталога, например, чтобы потом в цикле обработать каждый элемент каталога. В самых простых случаях достаточно метода os.listdir, который возвращает список файлов/каталогов в указанной директории. По умолчанию — текущая директория.
for filename in os.listdir(): print(filename, end=" ")
.ipynb_checkpoints about_12_and_so_on.ipynb about_python.ipynb argparse.ipynb custom_classes.ipynb custom_exceptions.ipynb decorators.ipynb dictionaries.ipynb dynamic_typing.ipynb exceptions.ipynb exercises1.ipynb exercises2.ipynb exercises3.ipynb files.ipynb filesystem.ipynb functions.ipynb garbage_collector.ipynb generators.ipynb if_for_range.ipynb inheritance.ipynb iterators.ipynb json.ipynb jupyter.ipynb LBYL_vs_EAFP.ipynb list_comprehensions.ipynb mutability.ipynb numbers_and_lists.ipynb operators_overloading.ipynb polymorphism.ipynb python_scripts.ipynb scripts_vs_modules.ipynb sequencies.ipynb tmp
Важно помнить, что согласно документации этот метод возвращает список файлов в произвольном порядке, т.е. он ни коим образом не отсортирован. Если требуется отсортировать их по названию, например, в алфавитном порядке, то можно воспользоваться встроенной функцией sorted. Практически во всех остальных случаях лучше выбрать os.scandir, которая не только возвращает содержимое каталога (тоже в произвольном порядке), но и метаинформацию о каждом файле.
Метод glob.glob модуля стандартной библиотеки glob позволяет фильтровать содержимое каталога на основе шаблона. В ячейке ниже демонстрируется, как можно найти все файлы в каталоге, которые начинаются с символа “a
”, а завершаются расширением “.ipynb
”.
import glob for filename in glob.glob("a*.ipynb"): print(filename)
about_12_and_so_on.ipynb about_python.ipynb argparse.ipynb
Создание, копирование, перемещение и удаление файлов и каталогов#
Метод os.mkdir создаёт каталог, но две особенности:
-
если такой каталог уже существует, то бросается исключение;
-
если родительского каталога не существует, то тоже бросается исключение.
Альтернативой является метод os.makedirs имеет опциональный параметр exist_ok
, который позволяет игнорировать ошибку, возникающую при попытке создать уже существующий каталог. Кроме того, если для создания указанного каталога, потребуется создать несколько директорий по пути, то они тоже будут созданы.
Таким образом метод os.mkdir более осторожный, т.к. он точно даст знать, если вы пытаетесь повторно создать директорию, а также если вы где-то ошиблись в пути, а метод os.makedirs более гибкий, позволяющий сократить объем кода, но если вы ошиблись при составлении желаемого пути (например, опечатались в имени одного каталога), то вы не получите никакого сообщения об ошибке и итоговая директория все равно будет создана.
Модуль стандартной библиотеки shutil содержит набор методов, имитирующих методы командной строки, что позволяет копировать файлы (методы shutil.copy, shutil.copy2 и shutil.copyfile), копировать директории с их содержимым (метод shutil.copytree), удалять директории (метод shutil.rmtree) и перемещать файлы или директории (метод shutil.move).
Удалять файлы можно методом os.remove.
-
Setting File Paths in Python
-
How to Set the File Path Using the (
\
) Character -
How to Set the File Path Using the Raw String Literals
-
How to Set the File Path Using the
os.path.join()
Function -
How to Set the File Path Using the
pathlib.Path()
Function -
How to Set Absolute Paths in Python
-
How to Set Relative Paths in Python
-
Conclusion
A file path is a string that specifies the unique location of a file or directory. Setting file paths in Python is a fundamental skill for working with files and directories.
Understanding how to specify paths correctly is crucial for reading, writing, or manipulating files. This tutorial will discuss how to set the path for a file in Python on Windows operating systems.
Setting File Paths in Python
Mostly, we are provided with the default path variable when we install Python. But sometimes, we have to set these variables manually, or if we want to set a different path, we have to do it manually.
To execute functions on files stored in our directories, it’s crucial to provide the program with the complete file path; a directory path often resembles "C:\Folder"
.
In this guide post, we explore best practices to overcome these nuances and ensure seamless file path handling in your Python scripts.
How to Set the File Path Using the (\
) Character
On Windows systems, the backslash (\
) is commonly used as the directory separator. We can use the (\\
) character in place of a single (\
) to provide the same path in Python.
Setting a file path in Python using the backslash (\
) character requires careful consideration due to its interpretation as an escape character in Python strings.
The syntax for this is shown below.
Below is an example demonstrating how to set a file path using the (\
) character.
Code Input:
folder = "documents"
filename = "example.txt"
file_path = "C:\\Users\\" + folder + "\\" + filename
print("File Path using the \ Character:", file_path)
Output:
In this example, we build a file path using the backslash (\
) character. Variables folder and filename are set to "documents"
and "example.txt"
.
Concatenating them in the file_path
string, starting with "C:\\Users\\"
, creates the complete file path. The output displays this constructed path, ensuring proper formatting for a Windows file path.
How to Set the File Path Using the Raw String Literals
We can use raw string literals to provide paths for the files as a raw string will treat these backslashes as a literal character.
To make a raw string, note that we have to write the (r
) character before the quotes for the string.
The syntax for using raw string literals is shown below.
Below is an example demonstrating how to set a file path using raw string literals.
Code Input:
folder = "documents"
filename = "example.txt"
file_path = r"C:\Users\Username" + "\\" + folder + r"\\" + filename
print("File Path using using raw string literal:", file_path)
Output:
In this example, we build a file path object using a raw string literal, indicated by the 'r'
prefix. The folder
variable is set to "documents"
, and the filename
variable is set to "example.txt"
.
By concatenating these variables within the file_path
string and using a raw string literal "C:\Users\Username\\"
as the base, we create the complete file path. The output then displays this constructed path, ensuring that backslashes are treated as literal characters, maintaining their intended role as separators in a Windows file path.
This approach helps avoid unintended interpretations of backslashes as escape characters within the string.
How to Set the File Path Using the os.path.join()
Function
We can also use the os.path()
function of the setting up the path to same directory. The advantage of using the path()
function is that we don’t need to specify the complete path for the local file, we have to provide the directory name and the file name.
This method automatically adapts to the correct configuration for the working directory based on the operating system you are using on your device. We have to use the join()
function to combine the directory and the root filename.
Code Input:
import os
print(os.path.join("C:", os.sep, "Users"))
Output:
In this example, we use the os.path.join()
method to create a file path. The components "C:"
, the default OS separator (os.sep
), and "Users"
are joined together.
The method ensures proper formatting of folder paths specific to the operating system. The output, C:\Users
, showcases a correctly constructed user file path for Windows.
How to Set the File Path Using the pathlib.Path()
Function
In Python 3.4 and above, we can use the Path()
function from the pathlib
module to specify the file paths in Python. Its use is similar to the os.path()
function.
Set the File Path in Python 3.4 Using the pathlib.Path()
Function
Code Input:
from pathlib import Path
print(Path("C:", "/", "Users"))
Output:
In this example, using the Path
class, we construct a file path object with components "C:"
, "/"
, and "Users"
. The output, C:\Users
, demonstrates the successful creation of a platform-independent path, enhancing code clarity and portability.
How to Set Absolute Paths in Python
Setting an absolute file path in Python involves specifying the complete and unambiguous location of a file within the file system. Below are examples using both the os.path module and the pathlib module, two commonly used approaches.
How to Set Absolute Path Using the os.path
Module
Code Input:
import os
# Specify folder and filename
folder = "documents"
filename = "example.txt"
# Set absolute path manually
absolute_path = os.path.join("C:/Users", folder, filename)
print("Absolute Path using os.path:", absolute_path)
Output:
How to Set Absolute Path Using the pathlib
Module
Code Input:
from pathlib import Path
# Specify folder and filename
folder = "documents"
filename = "example.txt"
# Set absolute path manually
absolute_path = Path("C:/Users") / folder / filename
print("Absolute Path using pathlib:", absolute_path)
Output:
How to Set Relative Paths in Python
Setting relative file paths is often necessary when you want to reference files or directories based on their position relative to the location of your Python script or the current working directory. It’s an important concept, especially when you need your code to be portable across different systems or when organizing files and folders within a project structure.
How to Set Relative Path Using the os.path
Module
Code Input:
import os
# Specify the relative path to a file or directory
relative_path = "documents/example.txt"
# Get the current working directory
current_directory = os.getcwd()
# Combine the current directory and the relative path
file_path = os.path.join(current_directory, relative_path)
print("Relative Path in Python:", file_path)
Output:
How to Set Relative Path Using the pathlib
Module
Code Input:
from pathlib import Path
# Specify the relative path to a file or directory
relative_path = "documents/example.txt"
# Get the current working directory
current_directory = Path.cwd()
# Combine the current directory and the relative path
file_path = current_directory / relative_path
print("Relative Path in Python:", file_path)
Output:
Conclusion
In the post in conclusion, this guide delves into the crucial skill of setting file paths in Python, specifically focusing on the Windows operating system. Starting with the fundamentals of file paths and the potential challenges associated with backslashes, it proceeds to demonstrate practical examples.
The guide explores the use of double backslashes, raw string literals, and the os.path.join()
and pathlib.Path()
functions for constructing file paths. Each method is illustrated with examples and outputs, showcasing how to set paths for such file in various scenarios.
Additionally, the guide extends its coverage to absolute file paths, showcasing approaches using both os.path
and pathlib
modules.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe